Introduction
The subject of delegating permissions in Active Directory for management of computer objects has been covered many times in many forums. I wanted to try to collect all that information as well as add some refinements of my own.
Rights vs. permissions
In the olden days, back when I was just a wee lad and Windows NT was new, the ability to join a computer to a domain was controller by a user right called Add workstations to domain. This user right is only valid on domain controllers. Any use who had this right could join computers to a Windows NT domain. The user right still exists and is in use even on Windows Server 2012 R2 Domain Controllers running Active Directory domains. When users join their computers to the domain using their own credentials they do it using this user right. It is, in fact, the only option available to them, since no regular users are granted any permissions over computer objects in Active Directory by default.
Back in the NT era this right was granted to the Users group and there was no limit to how many computers any give user could add to the domain. When Windows 2000 came along and with it Active Directory the user right was changed to apply to the Authenticated Users security principal and any one user could only add 10 computers to a domain by default. But the preferred way for Active Directory was to use permissions in the directory service to control object creation, modification and deletion…
Active Directory has a very fine grained permissions set allowing you to set permissions for objects as well as their properties. These permissions work the same way as the rest of the authorization model in Windows does by using Access Control Lists (ACL) with security principals and their permissions listed in individual Access Control Entries (ACE). Permissions can be granted anywhere in the hierarchy and inherited down to objects and containers. Granting permissions in Active Directory to someone or something is often called delegation. Computer objects are of course also included in these permissions and we can create much better delegation of control than we could with just a global user right.
We’ll cover three delegation of control scenarios regarding computer object management in this post:
- Allowing a security principal to join (add) a computer to a domain
- Allowing a security principal to join and re-join a computer to a domain
- Allowing a security principal to rename a computer in a domain
- Allowing a security principal to move computer objects in a domain
You can of course combine any of these.
Creating a computer object and changing its properties is what is required to join a computer to the domain. The container could be the Computers container or any other OU or container, including the domain itself but I do not recommend that.
When a computer joins an Active Directory domain without specifying a path, it is placed in the Computers container. The Computers container is not an OU and so it cannot have Group Policy Objects linked to it or have sub containers or OUs. If you are fine with all computer objects being created in this container you can delegate the permissions below to the Computers container
1. Allowing a security principal to join (add) a computer to a domain
First out is the most common scenario; join a computer to an Active Directory domain. As stated earlier it is not necessary to delegate this to regular users since the very few cases where they join their own computers to a domain should be covered by the Add workstation to domain user right. One exception to this is if you want to tighten down security and remove all security principals from this user right. In that case, if you still want to allow regular users to be able to join computers to a domain you have to delegate permissions to them. The more common case is that whatever deployment solution you use adds the computers to the domain. This is by far the best solution since very few users have any idea what a domain is.
A best practice is to create a service account used only for adding computers to the domain. This account should be clearly labeled, have a strong password and not have any other rights or permissions in you directory except the ability to join the domain. That being said the procedure below works for any security principal you want to delegate permissions to join the domain for.
- Identify the security principal that you want to delegate permissions for
This can be any security principal; user, group etc.
- Identify the container or OU where you want to allow users to manipulate computer objects
- Right click the container or OU you selected and select Delegate Control…
- The Delegation of Control Wizard opens, hit Next
- The Users or Groups window opens:
Select the security principal you want to grant permissions to, then hit Next again.
- The Tasks to Delegate window opens:
Select Create a custom task to delegate and hit Next
- The Active Directory Object Type window opens:
Select Only the following objects in the folder and select Computer objects, select Create selected objects in this folder and finally hit Next
- The Permissions window opens
Select Property-specific and select Read All Properties. This is actually redundant since this permissions are already granted to the Authenticated Users principal, but the delegation of control wizard will not let you continue without selecting something on this screen.
- Finally the Completing the Delegation of Control Wizard window opens showing you a summary of your actions. Hit Finish.
Delegation of Control Wizard Summary
You chose to delegate control of objects
in the following Active Directory folder:
saferoad.com/Computers
The groups, users, or computers to which you
have given control are:
Domain Join Account (SvcJoinComputerToDom@saferoad.com)
They have the following permissions:
Read All Properties
For the following object types:
Computer
The selected principals can now join computers to the domain.
2. Allowing a security principal to join and re-join a computer to a domain
The second scenario; allowing a principal to also re-join a computer to a domain requires some additional permissions. This is useful if you want to have a service account that can manage all computer accounts, also existing ones. System Center Configuration Manager for example requires these permissions.
- Identify the security principal that you want to delegate permissions for
- Identify the container or OU where you want to allow users to create and configure computer objects
- Right click the container or OU you selected and select Delegate Control…
- The Delegation of Control Wizard opens, hit Next
- The Users or Groups window opens:
Select the security principal you want to grant permissions to, then hit Next again.
- The Tasks to Delegate window opens:
Select Create a custom task to delegate and hit Next
- The Active Directory Object Type window opens:
Select Only the following objects in the folder and select Computer objects, select Create selected objects in this folder and Delete selected objects in this folder, and finally hit Next
- The Permissions window opens
Select Property-specific and select these individual permissions:
– Reset Password
– Read and write Account Restrictions
– Validated write to DNS host name
– Validated write to service principal name
- Finally the Completing the Delegation of Control Wizard window opens showing you a summary of your actions. Hit Finish.
Delegation of Control Wizard Summary
You chose to delegate control of objects
in the following Active Directory folder:
<domain>/Computers
The groups, users, or computers to which you
have given control are:
Domain Join Account (SvcJoinComputerToDom@<domain>)
They have the following permissions:
Reset password
Read and write account restrictions
Validated write to DNS host name
Validated write to service principal name
For the following object types:
Computer
The selected principals can now join computers to the domain as well as re-join computers when the computer account already exists.
3. Allowing a security principal to rename a computer in a domain
Here goes no. 3…
- Identify the security principal that you want to delegate permissions for
- Identify the container or OU where you want to allow users to create and configure computer objects
- Right click the container or OU you selected and select Delegate Control…
- The Delegation of Control Wizard opens, hit Next
- The Users or Groups window opens:
Select the security principal you want to grant permissions to, then hit Next again.
- The Tasks to Delegate window opens:
Select Create a custom task to delegate and hit Next
- The Active Directory Object Type window opens:
Select Only the following objects in the folder and select Computer objects and hit Next
- The Permissions window opens
Select Property-specific and select Write All Properties.
Scroll down an add these individual permissions as well:
– Validated write to DNS host name
– Validated write to service principal name
- Finally the Completing the Delegation of Control Wizard window opens showing you a summary of your actions. Hit Finish.
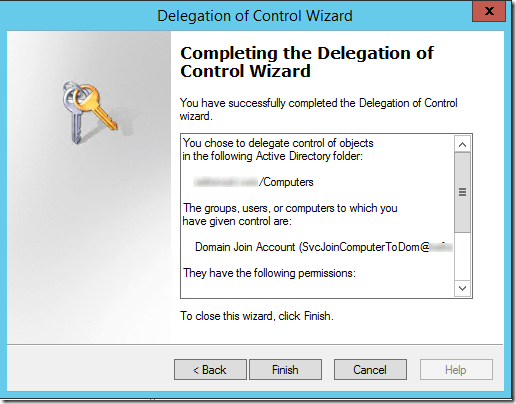
Delegation of Control Wizard Summary
You chose to delegate control of objects
in the following Active Directory folder:
saferoad.com/Computers
The groups, users, or computers to which you
have given control are:
Domain Join Account (SvcJoinComputerToDom@saferoad.com)
They have the following permissions:
Write All Properties
Validated write to DNS host name
Validated write to service principal name
For the following object types:
Computer
The selected principals can now rename a computer in a domain.
4. Allowing a security principal to move computer objects in a domain
The last one is a little more involved as it requires changing permissions on both the source container/OU and the destination. The destination container/OU requires the same permissions as in scenario 1 so set those. Follow these steps to configure the source container/OU:
- Identify the security principal that you want to delegate permissions for
- Identify the container or OU where you want to allow users to create and configure computer objects
- Right click the container or OU you selected and select Delegate Control…
- The Delegation of Control Wizard opens, hit Next
- The Users or Groups window opens:
Select the security principal you want to grant permissions to, then hit Next again.
- The Tasks to Delegate window opens:
Select Create a custom task to delegate and hit Next
- The Active Directory Object Type window opens:
Select Only the following objects in the folder, select Computer objects and , select Delete selected objects in this folders, hit Next
- The Permissions window opens
Select Property-specific and select Write All Properties.
- Finally the Completing the Delegation of Control Wizard window opens showing you a summary of your actions. Hit Finish.
Delegation of Control Wizard Summary
You chose to delegate control of objects
in the following Active Directory folder:
saferoad.com/Computers
The groups, users, or computers to which you
have given control are:
Domain Join Account (SvcJoinComputerToDom@saferoad.com)
They have the following permissions:
Write All Properties
For the following object types:
Computer
More information
There is an interesting distinction between joining a domain by exercising the user right Add workstation to the domain and using delegated permissions. If you join by the user right the owner of the resulting computer object is the Domain Administrators group, but if you join by delegated permissions the owner is the user who actually performed the join. Also if a principal has both the user right and delegated permissions, delegated permissions take precedent and are used to join the computer to the domain. A recommended best practice her would be to remove all principals from the user right and just rely on permissions. You do that by applying a policy to your domain controllers where no principal has the user right:

The location of the user right is:
Computer Configuration\Policies\Windows Settings\Security Settings\Local Policies\User Rights Assignment
You could also increase the default quota of 10 computer accounts added to the domain per user, but I do not recommend that. I essence you are copying the settings from Windows NT to your Active Directory domain and do not take advanced of the advanced delegation model in Active Directory. For completion here is how to change the quota:
From any editor capable of displaying and changing individual attributes of Active Directory objects; display the properties of the domain NC. Locate the ms-DS-MachineAccountQuota property and change it to your desired value:

Links
Here are a few links for further info: